Plant API Documentation
Join our community of coders, get help & ask questions in a collaborative environment!😄
OVER 10,000+ SPECIES OF PLANTS AVAILABLE
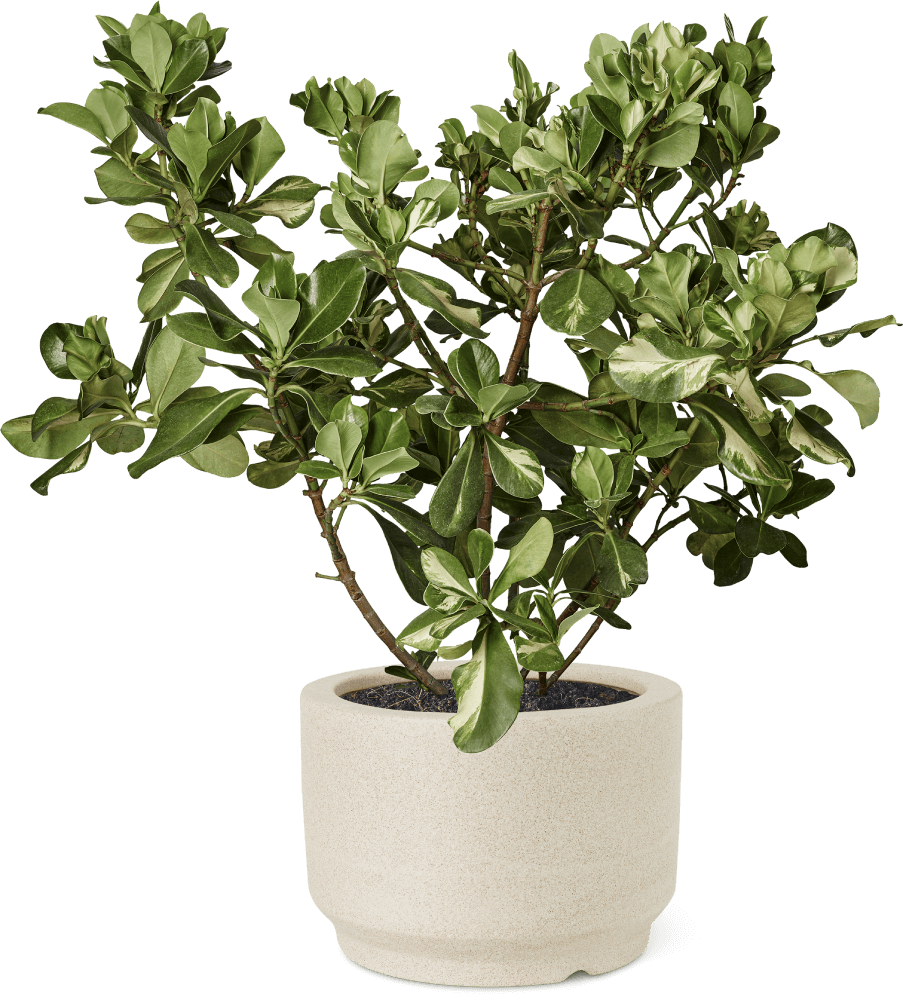
GET BOTANICAL DATA FOR PLANTS
Our plant/botanic API is a custom-built RESTful API designed to help developers/farmers/botanist quickly and easily build agriculture/plant-related services and applications. It provides access to a wealth of plant-based information, including species, care guides, growth stages, images, hardiness zones and much more, making it an ideal choice to create agriculture/plant-related applications and services. Our API also provides many niche farming/botany/botanical resources for varieties like crops, tropical houseplants, sustainable outdoor plants, non-poisonous edible fruits/berries and medical herbs
GET https://perenual.com/api/v2/species-list?key=[YOUR-API-KEY]
<!-- Example JSON Response --> { "data": [ { "id": 1, "common_name": "European Silver Fir", "scientific_name": [ "Abies alba" ], "other_name": [ "Common Silver Fir" ], "family": null, "hybrid": null, "authority": null, "subspecies": null, "cultivar": null, "variety": null, "species_epithet": "alba", "genus": "Abies", "default_image": { "image_id": 9, "license": 5, "license_name": "Attribution-ShareAlike License", "license_url": "https://creativecommons.org/licenses/by-sa/2.0/", "original_url": "https://perenual.com/storage/species_image/2_abies_alba_pyramidalis/og/49255769768_df55596553_b.jpg", "regular_url": "https://perenual.com/storage/species_image/2_abies_alba_pyramidalis/regular/49255769768_df55596553_b.jpg", "medium_url": "https://perenual.com/storage/species_image/2_abies_alba_pyramidalis/medium/49255769768_df55596553_b.jpg", "small_url": "https://perenual.com/storage/species_image/2_abies_alba_pyramidalis/small/49255769768_df55596553_b.jpg", "thumbnail": "https://perenual.com/storage/species_image/2_abies_alba_pyramidalis/thumbnail/49255769768_df55596553_b.jpg" } }, { "id": 2, "common_name": "Pyramidalis Silver Fir", "scientific_name": [ "Abies alba 'Pyramidalis'" ], "other_name": null, "family": null, "hybrid": null, "authority": null, "subspecies": null, "cultivar": "Pyramidalis", "variety": null, "species_epithet": "alba", "genus": "Abies", "default_image": { "image_id": 9, "license": 5, "license_name": "Attribution-ShareAlike License", "license_url": "https://creativecommons.org/licenses/by-sa/2.0/", "original_url": "https://perenual.com/storage/species_image/2_abies_alba_pyramidalis/og/49255769768_df55596553_b.jpg", "regular_url": "https://perenual.com/storage/species_image/2_abies_alba_pyramidalis/regular/49255769768_df55596553_b.jpg", "medium_url": "https://perenual.com/storage/species_image/2_abies_alba_pyramidalis/medium/49255769768_df55596553_b.jpg", "small_url": "https://perenual.com/storage/species_image/2_abies_alba_pyramidalis/small/49255769768_df55596553_b.jpg", "thumbnail": "https://perenual.com/storage/species_image/2_abies_alba_pyramidalis/thumbnail/49255769768_df55596553_b.jpg" } } ... ], "to": 30, "per_page": 30, "current_page": 1, "from": 1, "last_page": 405, "total": 10104 }
// Example React Component import React, { useState, useEffect } from 'react'; import axios from 'axios'; function App() { const [data, setData] = useState(null); useEffect(() => { async function fetchData() { try { const response = await axios.get(`https://perenual.com/api/v2/species-list?key=[YOUR-API-KEY]`); setData(response.data); } catch (error) { console.error("Error fetching data:", error); } } fetchData(); }, []); return ( <div> <h1>API Data</h1> <pre>{JSON.stringify(data, null, 2)}</pre> </div> ); } export default App;
// Example Next.js Component import { useState } from 'react'; import axios from 'axios'; export default function Home() { const [response, setResponse] = useState(null); const Request = async () => { try { const result = await axios.get(`https://perenual.com/api/v2/species-list?key=[YOUR-API-KEY]`, { someData: 'test' }); // Replace the data with your actual payload setResponse(result.data); } catch (error) { console.error("Error occured:", error); setResponse(error.message); } }; return ( <div> <button onClick={Request}>Upload Image</button> {response && <div>Response: {JSON.stringify(response)}</div>} </div> ); }
// Example Javascript Component var requestOptions = { method: '', redirect: 'follow' }; fetch(`https://perenual.com/api/v2/species-list?key=[YOUR-API-KEY]`, requestOptions) .then(response => response.text()) .then(result => console.log(result)) .catch(error => console.log('error', error));
// Example Python Component import requests url = "https://perenual.com/api/v2/species-list?key=[YOUR-API-KEY]" payload = {} headers = {} response = requests.request("", url, headers=headers, data=payload) print(response.text)
// Example PHP Component <?php $client = new GuzzleHttp\Client(); $url = "https://perenual.com/api/v2/species-list?key=[YOUR-API-KEY]"; $response = $client->request('', $url); echo $response->getBody(); ?>
// Example NodeJS Component const axios = require('axios'); const url = `https://perenual.com/api/v2/species-list?key=[YOUR-API-KEY]`; axios.get(url) .then((response) => { console.log(JSON.stringify(response.data)); }) .catch((error) => { console.log(error); });
// Example Ruby Component require "uri" require "net/http" url = URI("https://perenual.com/api/v2/species-list?key=[YOUR-API-KEY]") http = Net::HTTP.new(url.host, url.port); request = Net::HTTP::.new(url) response = http.request(request) puts response.read_body
// Example Java Component Unirest.setTimeouts(0, 0); HttpResponse
response = Unirest.get("https://perenual.com/api/v2/species-list?key=[YOUR-API-KEY]") .asString(); // Example C# Component var client = new HttpClient(); var url = $"https://perenual.com/api/v2/species-list?key=[YOUR-API-KEY]"; var response = await client.Async(url); response.EnsureSuccessStatusCode(); Console.WriteLine(await response.Content.ReadAsStringAsync());
// Example Go Component package main import ( "fmt" "net/http" "io/ioutil" ) func main() { url := "https://perenual.com/api/v2/species-list?key=[YOUR-API-KEY]" client := &http.Client {} req, err := http.NewRequest("", url, nil) if err != nil { fmt.Println(err) return } res, err := client.Do(req) if err != nil { fmt.Println(err) return } defer res.Body.Close() body, err := ioutil.ReadAll(res.Body) if err != nil { fmt.Println(err) return } fmt.Println(string(body)) }
// Example cURL Component curl --location 'https://perenual.com/api/v2/species-list?key=[YOUR-API-KEY]'
// Example Swift Component let urlString = "https://perenual.com/api/v2/species-list?key=[YOUR-API-KEY]" guard let url = URL(string: urlString) else { return } var request = URLRequest(url: url, timeoutInterval: Double.infinity) request.httpMethod = "" let task = URLSession.shared.dataTask(with: request) { data, response, error in guard let data = data else { print(String(describing: error)) return } print(String(data: data, encoding: .utf8)!) } task.resume()
// Example Dart Component var request = http.Request('', Uri.parse('https://perenual.com/api/v2/species-list?key=[YOUR-API-KEY]')); http.StreamedResponse response = await request.send(); if (response.statusCode == 200) { print(await response.stream.bytesToString()); } else { print(response.reasonPhrase); }
// Example Kotlin Component val client = OkHttpClient() val request = Request.Builder() .url("https://perenual.com/api/v2/species-list?key=[YOUR-API-KEY]") .post(RequestBody.create(null, ByteArray(0))) .build() val response = client.newCall(request).execute()
GET https://perenual.com/api/v2/species/details/[ID]?key=[YOUR-API-KEY]
<!-- Example JSON Response --> { "id": 1, "common_name": "European Silver Fir", "scientific_name": [ "Abies alba" ], "other_name": [ "Common Silver Fir" ], "family": "", "origin": null, "type": "tree", "dimensions": { "type": null, "min_value": 1, "max_value": 1.5, "unit": "feet" }, "cycle": "Perennial", "watering": "Frequent", "watering_general_benchmark": { "value": 5-7, "unit": "days" }, "plant_anatomy": [ { "part": "leaves", "color": [ "dark-green" ] }, { "part": "branches", "color": [ "dark-brown" ] }, { "part": "twigs", "color": [ "brown" ] } ], "sunlight": [ "Part shade" ], "pruning_month": [ "March", "April" ], "pruning_count": { "amount": 1, "interval": "yearly" }, "seeds": 0, "attracts":[ "bees", "birds", "rabbits" ], "propagation":[ "seed", "cutting" ], "hardiness": { "min": "7", "max": "7" }, "hardiness_location": { "full_url": "https://perenual.com/api/hardiness-map-sample?map=h&key=[YOUR-API-KEY]", "full_iframe": "<iframe src='https://perenual.com/api/hardiness-map-sample?map=1-13&key=[YOUR-API-KEY]' width=1000 height=550 ></iframe>" }, "flowers": true, "flowering_season": "Spring", "sunlight": [ "full sun", "part shade" ], "soil": [], "pest_susceptibility": null, "cones": true, "fruits": false, "edible_fruit": false, "fruiting_season": null, "harvest_season": null, "harvest_method": "cutting", "leaf": true, "edible_leaf": false, "growth_rate": "High", "maintenance": "Low", "medicinal": true, "poisonous_to_humans": false, "poisonous_to_pets": false, "drought_tolerant": false, "salt_tolerant": false, "thorny": false, "invasive": false, "rare": false, "tropical": false, "cuisine": false, "indoor": false, "care_level": "Medium", "description": "Amazing garden plant that is sure to capture attention...", "default_image": { "image_id": 9, "license": 5, "license_name": "Attribution-ShareAlike License", "license_url": "https://creativecommons.org/licenses/by-sa/2.0/", "original_url": "https://perenual.com/storage/species_image/2678_abies_alba_pyramidalis/og/49255768_df596553_b.jpg", "regular_url": "https://perenual.com/storage/species_image/2678_abies_alba_pyramidalis/regular/4925769768f55596553_b.jpg", "medium_url": "https://perenual.com/storage/species_image/882_abies_alba_pyramidalis/medium/4925576768_f55596553_b.jpg", "small_url": "https://perenual.com/storage/species_image/2678_abies_alba_pyramidalis/small/492557668_df55596553_b.jpg", "thumbnail": "https://perenual.com/storage/species_image/2786_abies_alba_pyramidalis/thumbnail/4929768_df55596553_b.jpg" }, "other_images":[{ "image_id": 9, "license": 5, "license_name": "Attribution-ShareAlike License", "license_url": "https://creativecommons.org/licenses/by-sa/2.0/", "original_url": "https://perenual.com/storage/species_image/22_abies_alba_pyramidalis/og/4769768_df55596553_b.jpg", "regular_url": "https://perenual.com/storage/species_image/22_abies_alba_pyramidalis/regular/492557768_df55596553_b.jpg", "medium_url": "https://perenual.com/storage/species_image/22_abies_alba_pyramidalis/medium/49259768_df55596553_b.jpg", "small_url": "https://perenual.com/storage/species_image/21_abies_alba_pyramidalis/small/492557668_df556553_b.jpg", "thumbnail": "https://perenual.com/storage/species_image/21_abies_alba_pyramidalis/thumbnail/255768_df55553_b.jpg" }]..., "xWateringQuality": [ "Rainwater", "Distilled Water", "Reverse Osmosis Water", "Spring Water", "Well Water", "Aquarium Water", "Pond/Lake Water" ], "xWateringPeriod": [ "Morning", "Evening" ], "xWateringAvgVolumeRequirement": [], "xWateringDepthRequirement": [], "xWateringBasedTemperature": { "unit": "celcius", "min": 10, "max": 20 }, "xWateringPhLevel": { "min": 5.5, "max": 6.5 }, "xSunlightDuration": { "min": "6", "max": "", "unit": "hours" } }
// Example React Component import React, { useState, useEffect } from 'react'; import axios from 'axios'; function App() { const [data, setData] = useState(null); useEffect(() => { async function fetchData() { try { const response = await axios.get(`https://perenual.com/api/v2/species/details/[ID]?key=[YOUR-API-KEY]`); setData(response.data); } catch (error) { console.error("Error fetching data:", error); } } fetchData(); }, []); return ( <div> <h1>API Data</h1> <pre>{JSON.stringify(data, null, 2)}</pre> </div> ); } export default App;
// Example Next.js Component import { useState } from 'react'; import axios from 'axios'; export default function Home() { const [response, setResponse] = useState(null); const Request = async () => { try { const result = await axios.get(`https://perenual.com/api/v2/species/details/[ID]?key=[YOUR-API-KEY]`, { someData: 'test' }); // Replace the data with your actual payload setResponse(result.data); } catch (error) { console.error("Error occured:", error); setResponse(error.message); } }; return ( <div> <button onClick={Request}>Upload Image</button> {response && <div>Response: {JSON.stringify(response)}</div>} </div> ); }
// Example Javascript Component var requestOptions = { method: '', redirect: 'follow' }; fetch(`https://perenual.com/api/v2/species/details/[ID]?key=[YOUR-API-KEY]`, requestOptions) .then(response => response.text()) .then(result => console.log(result)) .catch(error => console.log('error', error));
// Example Python Component import requests url = "https://perenual.com/api/v2/species/details/[ID]?key=[YOUR-API-KEY]" payload = {} headers = {} response = requests.request("", url, headers=headers, data=payload) print(response.text)
// Example PHP Component <?php $client = new GuzzleHttp\Client(); $url = "https://perenual.com/api/v2/species/details/[ID]?key=[YOUR-API-KEY]"; $response = $client->request('', $url); echo $response->getBody(); ?>
// Example NodeJS Component const axios = require('axios'); const url = `https://perenual.com/api/v2/species/details/[ID]?key=[YOUR-API-KEY]`; axios.get(url) .then((response) => { console.log(JSON.stringify(response.data)); }) .catch((error) => { console.log(error); });
// Example Ruby Component require "uri" require "net/http" url = URI("https://perenual.com/api/v2/species/details/[ID]?key=[YOUR-API-KEY]") http = Net::HTTP.new(url.host, url.port); request = Net::HTTP::.new(url) response = http.request(request) puts response.read_body
// Example Java Component Unirest.setTimeouts(0, 0); HttpResponse
response = Unirest.get("https://perenual.com/api/v2/species/details/[ID]?key=[YOUR-API-KEY]") .asString(); // Example C# Component var client = new HttpClient(); var url = $"https://perenual.com/api/v2/species/details/[ID]?key=[YOUR-API-KEY]"; var response = await client.Async(url); response.EnsureSuccessStatusCode(); Console.WriteLine(await response.Content.ReadAsStringAsync());
// Example Go Component package main import ( "fmt" "net/http" "io/ioutil" ) func main() { url := "https://perenual.com/api/v2/species/details/[ID]?key=[YOUR-API-KEY]" client := &http.Client {} req, err := http.NewRequest("", url, nil) if err != nil { fmt.Println(err) return } res, err := client.Do(req) if err != nil { fmt.Println(err) return } defer res.Body.Close() body, err := ioutil.ReadAll(res.Body) if err != nil { fmt.Println(err) return } fmt.Println(string(body)) }
// Example cURL Component curl --location 'https://perenual.com/api/v2/species/details/[ID]?key=[YOUR-API-KEY]'
// Example Swift Component let urlString = "https://perenual.com/api/v2/species/details/[ID]?key=[YOUR-API-KEY]" guard let url = URL(string: urlString) else { return } var request = URLRequest(url: url, timeoutInterval: Double.infinity) request.httpMethod = "" let task = URLSession.shared.dataTask(with: request) { data, response, error in guard let data = data else { print(String(describing: error)) return } print(String(data: data, encoding: .utf8)!) } task.resume()
// Example Dart Component var request = http.Request('', Uri.parse('https://perenual.com/api/v2/species/details/[ID]?key=[YOUR-API-KEY]')); http.StreamedResponse response = await request.send(); if (response.statusCode == 200) { print(await response.stream.bytesToString()); } else { print(response.reasonPhrase); }
// Example Kotlin Component val client = OkHttpClient() val request = Request.Builder() .url("https://perenual.com/api/v2/species/details/[ID]?key=[YOUR-API-KEY]") .post(RequestBody.create(null, ByteArray(0))) .build() val response = client.newCall(request).execute()
GET https://perenual.com/api/pest-disease-list?key=[YOUR-API-KEY]
<!-- Example JSON Response --> { "data": [ { "id": 2, "common_name": "Fungi Nuisance", "scientific_name": " Panaeolus foenisecii/", "other_name": [ "Nuisance fungi" ], "family": null, "description": null, "solution": null, "host": [ "all lawn grasses" ], "images": [ { "license": 45, "license_name": "Attribution-ShareAlike 3.0 Unported (CC BY-SA 3.0)", "license_url": "https://creativecommons.org/licenses/by-sa/3.0/deed.en", "original_url": "https://perenual.com/storage/species_disease/2__/og/Panaeolus_foenisecii_124316833.jpg", "regular_url": "https://perenual.com/storage/species_disease/2__/regular/Panaeolus_foenisecii_124316833.jpg", "medium_url": "https://perenual.com/storage/species_disease/2__/medium/Panaeolus_foenisecii_124316833.jpg", "small_url": "https://perenual.com/storage/species_disease/2__/small/Panaeolus_foenisecii_124316833.jpg", "thumbnail": "https://perenual.com/storage/species_disease/2__/thumbnail/Panaeolus_foenisecii_124316833.jpg" } ] } ... ], "to": 30, "per_page": 30, "current_page": 1, "from": 1, "last_page": 8, "total": 239 }
// Example React Component import React, { useState, useEffect } from 'react'; import axios from 'axios'; function App() { const [data, setData] = useState(null); useEffect(() => { async function fetchData() { try { const response = await axios.get(`https://perenual.com/api/pest-disease-list?key=[YOUR-API-KEY]`); setData(response.data); } catch (error) { console.error("Error fetching data:", error); } } fetchData(); }, []); return ( <div> <h1>API Data</h1> <pre>{JSON.stringify(data, null, 2)}</pre> </div> ); } export default App;
// Example Next.js Component import { useState } from 'react'; import axios from 'axios'; export default function Home() { const [response, setResponse] = useState(null); const Request = async () => { try { const result = await axios.get(`https://perenual.com/api/pest-disease-list?key=[YOUR-API-KEY]`, { someData: 'test' }); // Replace the data with your actual payload setResponse(result.data); } catch (error) { console.error("Error occured:", error); setResponse(error.message); } }; return ( <div> <button onClick={Request}>Upload Image</button> {response && <div>Response: {JSON.stringify(response)}</div>} </div> ); }
// Example Javascript Component var requestOptions = { method: '', redirect: 'follow' }; fetch(`https://perenual.com/api/pest-disease-list?key=[YOUR-API-KEY]`, requestOptions) .then(response => response.text()) .then(result => console.log(result)) .catch(error => console.log('error', error));
// Example Python Component import requests url = "https://perenual.com/api/pest-disease-list?key=[YOUR-API-KEY]" payload = {} headers = {} response = requests.request("", url, headers=headers, data=payload) print(response.text)
// Example PHP Component <?php $client = new GuzzleHttp\Client(); $url = "https://perenual.com/api/pest-disease-list?key=[YOUR-API-KEY]"; $response = $client->request('', $url); echo $response->getBody(); ?>
// Example NodeJS Component const axios = require('axios'); const url = `https://perenual.com/api/pest-disease-list?key=[YOUR-API-KEY]`; axios.get(url) .then((response) => { console.log(JSON.stringify(response.data)); }) .catch((error) => { console.log(error); });
// Example Ruby Component require "uri" require "net/http" url = URI("https://perenual.com/api/pest-disease-list?key=[YOUR-API-KEY]") http = Net::HTTP.new(url.host, url.port); request = Net::HTTP::.new(url) response = http.request(request) puts response.read_body
// Example Java Component Unirest.setTimeouts(0, 0); HttpResponse
response = Unirest.get("https://perenual.com/api/pest-disease-list?key=[YOUR-API-KEY]") .asString(); // Example C# Component var client = new HttpClient(); var url = $"https://perenual.com/api/pest-disease-list?key=[YOUR-API-KEY]"; var response = await client.Async(url); response.EnsureSuccessStatusCode(); Console.WriteLine(await response.Content.ReadAsStringAsync());
// Example Go Component package main import ( "fmt" "net/http" "io/ioutil" ) func main() { url := "https://perenual.com/api/pest-disease-list?key=[YOUR-API-KEY]" client := &http.Client {} req, err := http.NewRequest("", url, nil) if err != nil { fmt.Println(err) return } res, err := client.Do(req) if err != nil { fmt.Println(err) return } defer res.Body.Close() body, err := ioutil.ReadAll(res.Body) if err != nil { fmt.Println(err) return } fmt.Println(string(body)) }
// Example cURL Component curl --location 'https://perenual.com/api/pest-disease-list?key=[YOUR-API-KEY]'
// Example Swift Component let urlString = "https://perenual.com/api/pest-disease-list?key=[YOUR-API-KEY]" guard let url = URL(string: urlString) else { return } var request = URLRequest(url: url, timeoutInterval: Double.infinity) request.httpMethod = "" let task = URLSession.shared.dataTask(with: request) { data, response, error in guard let data = data else { print(String(describing: error)) return } print(String(data: data, encoding: .utf8)!) } task.resume()
// Example Dart Component var request = http.Request('', Uri.parse('https://perenual.com/api/pest-disease-list?key=[YOUR-API-KEY]')); http.StreamedResponse response = await request.send(); if (response.statusCode == 200) { print(await response.stream.bytesToString()); } else { print(response.reasonPhrase); }
// Example Kotlin Component val client = OkHttpClient() val request = Request.Builder() .url("https://perenual.com/api/pest-disease-list?key=[YOUR-API-KEY]") .post(RequestBody.create(null, ByteArray(0))) .build() val response = client.newCall(request).execute()
GET https://perenual.com/api/species-care-guide-list?key=[YOUR-API-KEY]
<!-- Example JSON Response --> { "data": [ { "id": 4, "species_id": 1, "common_name": "European Silver Fir", "scientific_name": [ "Abies alba bra2", "Abies alba zas" ], "section": [ { "id": 1, "type": "sunlight", "description": "Sunlight is the most important environmental factor controlling the growth and health of European silver fir (Abies alba). This species naturally grows in open, sunny habitats and is adapted to full sunlight or partial shade. Without adequate sunlight, the needles of a silver fir will fail to produce adequate amounts of chlorophyll, a key determinant of essential photosynthesis.\n\nWhen exposed to sunlight, the foliage of a European silver fir is usually thick, dense and lush. The needles are usually a deep green color, although in some areas the needles may take on a bluish hue. Sun-exposed needles are typically more abundant and tightly packed than those in more shaded areas. Furthermore, needles of silver fir trees normally grow more rapidly when exposed to direct sunlight.\n\nThe bark of a European silver fir exposed to direct sunlight typically has a more rugged and weathered appearance. Sun-exposed bark may contain lichens and moss, which act as a natural form of sun protection. In areas where the silver fir is shaded, the bark is typically smoother and lighter in color.\n\nSunlight is essential for the optimal growth and health of a European silver fir. Although this species can survive in both full sun and partial shade, it typically performs best when exposed to adequate sunlight." }, { "id": 3, "type": "watering", "description": "Watering European silver fir trees is essential for them to stay healthy. It is important to provide them with regular watering, especially during their first growing season, as they need to establish a good root system. A weekly deep watering is all they need, but they should be more frequently watered during periods of drought and heat. The soil should always be kept moist but never soggy.\n\nIf they are planted in a container, they should be watered more frequently as they can dry out quickly in containers. The soil should be kept moist but never overly wet. Overwatering can lead to root rot, so be sure not to overwater. When watering a container grown European Silver Fir, wait for the top 1-2 inches of soil to dry out before watering again.\n\nMulching around a European silver fir is a great way to help with water retention and help the soil stay moist. An organic mulch, such as wood chips or shredded bark, can help to keep the soil from drying out and also help reduce the amount of time spent watering. It also helps suppress weeds and keeps the roots protected from extreme temperatures.\n\nOverall, regular and consistent watering is important for young European silver fir trees to help them grow and thrive." } ] } ], "to": 2, "per_page": 30, "current_page": 1, "from": 1, "last_page": 1, "total": 2 }
// Example React Component import React, { useState, useEffect } from 'react'; import axios from 'axios'; function App() { const [data, setData] = useState(null); useEffect(() => { async function fetchData() { try { const response = await axios.get(`https://perenual.com/api/species-care-guide-list?key=[YOUR-API-KEY]`); setData(response.data); } catch (error) { console.error("Error fetching data:", error); } } fetchData(); }, []); return ( <div> <h1>API Data</h1> <pre>{JSON.stringify(data, null, 2)}</pre> </div> ); } export default App;
// Example Next.js Component import { useState } from 'react'; import axios from 'axios'; export default function Home() { const [response, setResponse] = useState(null); const Request = async () => { try { const result = await axios.get(`https://perenual.com/api/species-care-guide-list?key=[YOUR-API-KEY]`, { someData: 'test' }); // Replace the data with your actual payload setResponse(result.data); } catch (error) { console.error("Error occured:", error); setResponse(error.message); } }; return ( <div> <button onClick={Request}>Upload Image</button> {response && <div>Response: {JSON.stringify(response)}</div>} </div> ); }
// Example Javascript Component var requestOptions = { method: '', redirect: 'follow' }; fetch(`https://perenual.com/api/species-care-guide-list?key=[YOUR-API-KEY]`, requestOptions) .then(response => response.text()) .then(result => console.log(result)) .catch(error => console.log('error', error));
// Example Python Component import requests url = "https://perenual.com/api/species-care-guide-list?key=[YOUR-API-KEY]" payload = {} headers = {} response = requests.request("", url, headers=headers, data=payload) print(response.text)
// Example PHP Component <?php $client = new GuzzleHttp\Client(); $url = "https://perenual.com/api/species-care-guide-list?key=[YOUR-API-KEY]"; $response = $client->request('', $url); echo $response->getBody(); ?>
// Example NodeJS Component const axios = require('axios'); const url = `https://perenual.com/api/species-care-guide-list?key=[YOUR-API-KEY]`; axios.get(url) .then((response) => { console.log(JSON.stringify(response.data)); }) .catch((error) => { console.log(error); });
// Example Ruby Component require "uri" require "net/http" url = URI("https://perenual.com/api/species-care-guide-list?key=[YOUR-API-KEY]") http = Net::HTTP.new(url.host, url.port); request = Net::HTTP::.new(url) response = http.request(request) puts response.read_body
// Example Java Component Unirest.setTimeouts(0, 0); HttpResponse
response = Unirest.get("https://perenual.com/api/species-care-guide-list?key=[YOUR-API-KEY]") .asString(); // Example C# Component var client = new HttpClient(); var url = $"https://perenual.com/api/species-care-guide-list?key=[YOUR-API-KEY]"; var response = await client.Async(url); response.EnsureSuccessStatusCode(); Console.WriteLine(await response.Content.ReadAsStringAsync());
// Example Go Component package main import ( "fmt" "net/http" "io/ioutil" ) func main() { url := "https://perenual.com/api/species-care-guide-list?key=[YOUR-API-KEY]" client := &http.Client {} req, err := http.NewRequest("", url, nil) if err != nil { fmt.Println(err) return } res, err := client.Do(req) if err != nil { fmt.Println(err) return } defer res.Body.Close() body, err := ioutil.ReadAll(res.Body) if err != nil { fmt.Println(err) return } fmt.Println(string(body)) }
// Example cURL Component curl --location 'https://perenual.com/api/species-care-guide-list?key=[YOUR-API-KEY]'
// Example Swift Component let urlString = "https://perenual.com/api/species-care-guide-list?key=[YOUR-API-KEY]" guard let url = URL(string: urlString) else { return } var request = URLRequest(url: url, timeoutInterval: Double.infinity) request.httpMethod = "" let task = URLSession.shared.dataTask(with: request) { data, response, error in guard let data = data else { print(String(describing: error)) return } print(String(data: data, encoding: .utf8)!) } task.resume()
// Example Dart Component var request = http.Request('', Uri.parse('https://perenual.com/api/species-care-guide-list?key=[YOUR-API-KEY]')); http.StreamedResponse response = await request.send(); if (response.statusCode == 200) { print(await response.stream.bytesToString()); } else { print(response.reasonPhrase); }
// Example Kotlin Component val client = OkHttpClient() val request = Request.Builder() .url("https://perenual.com/api/species-care-guide-list?key=[YOUR-API-KEY]") .post(RequestBody.create(null, ByteArray(0))) .build() val response = client.newCall(request).execute()
GET https://perenual.com/api/hardiness-map?species_id=[ID]&key=[YOUR-API-KEY]
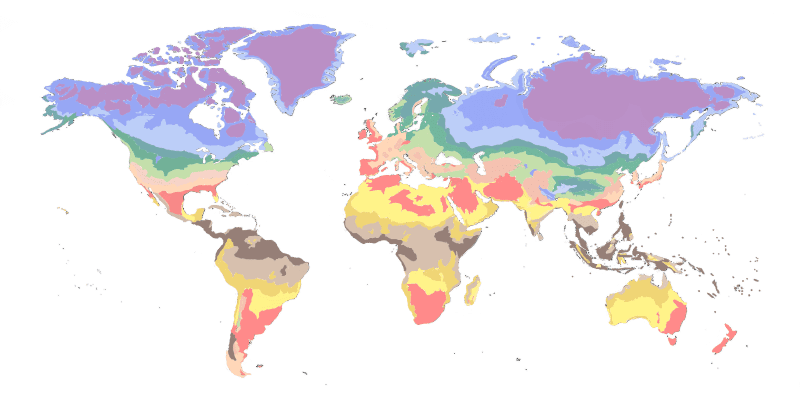
// Example React Component import React, { useState, useEffect } from 'react'; import axios from 'axios'; function App() { const [data, setData] = useState(null); useEffect(() => { async function fetchData() { try { const response = await axios.get(`https://perenual.com/api/hardiness-map?species_id=[ID]&key=[YOUR-API-KEY]`); setData(response.data); } catch (error) { console.error("Error fetching data:", error); } } fetchData(); }, []); return ( <div> <h1>API Data</h1> <pre>{JSON.stringify(data, null, 2)}</pre> </div> ); } export default App;
// Example Next.js Component import { useState } from 'react'; import axios from 'axios'; export default function Home() { const [response, setResponse] = useState(null); const Request = async () => { try { const result = await axios.get(`https://perenual.com/api/hardiness-map?species_id=[ID]&key=[YOUR-API-KEY]`, { someData: 'test' }); // Replace the data with your actual payload setResponse(result.data); } catch (error) { console.error("Error occured:", error); setResponse(error.message); } }; return ( <div> <button onClick={Request}>Upload Image</button> {response && <div>Response: {JSON.stringify(response)}</div>} </div> ); }
// Example Javascript Component var requestOptions = { method: '', redirect: 'follow' }; fetch(`https://perenual.com/api/hardiness-map?species_id=[ID]&key=[YOUR-API-KEY]`, requestOptions) .then(response => response.text()) .then(result => console.log(result)) .catch(error => console.log('error', error));
// Example Python Component import requests url = "https://perenual.com/api/hardiness-map?species_id=[ID]&key=[YOUR-API-KEY]" payload = {} headers = {} response = requests.request("", url, headers=headers, data=payload) print(response.text)
// Example PHP Component <?php $client = new GuzzleHttp\Client(); $url = "https://perenual.com/api/hardiness-map?species_id=[ID]&key=[YOUR-API-KEY]"; $response = $client->request('', $url); echo $response->getBody(); ?>
// Example NodeJS Component const axios = require('axios'); const url = `https://perenual.com/api/hardiness-map?species_id=[ID]&key=[YOUR-API-KEY]`; axios.get(url) .then((response) => { console.log(JSON.stringify(response.data)); }) .catch((error) => { console.log(error); });
// Example Ruby Component require "uri" require "net/http" url = URI("https://perenual.com/api/hardiness-map?species_id=[ID]&key=[YOUR-API-KEY]") http = Net::HTTP.new(url.host, url.port); request = Net::HTTP::.new(url) response = http.request(request) puts response.read_body
// Example Java Component Unirest.setTimeouts(0, 0); HttpResponse
response = Unirest.get("https://perenual.com/api/hardiness-map?species_id=[ID]&key=[YOUR-API-KEY]") .asString(); // Example C# Component var client = new HttpClient(); var url = $"https://perenual.com/api/hardiness-map?species_id=[ID]&key=[YOUR-API-KEY]"; var response = await client.Async(url); response.EnsureSuccessStatusCode(); Console.WriteLine(await response.Content.ReadAsStringAsync());
// Example Go Component package main import ( "fmt" "net/http" "io/ioutil" ) func main() { url := "https://perenual.com/api/hardiness-map?species_id=[ID]&key=[YOUR-API-KEY]" client := &http.Client {} req, err := http.NewRequest("", url, nil) if err != nil { fmt.Println(err) return } res, err := client.Do(req) if err != nil { fmt.Println(err) return } defer res.Body.Close() body, err := ioutil.ReadAll(res.Body) if err != nil { fmt.Println(err) return } fmt.Println(string(body)) }
// Example cURL Component curl --location 'https://perenual.com/api/hardiness-map?species_id=[ID]&key=[YOUR-API-KEY]'
// Example Swift Component let urlString = "https://perenual.com/api/hardiness-map?species_id=[ID]&key=[YOUR-API-KEY]" guard let url = URL(string: urlString) else { return } var request = URLRequest(url: url, timeoutInterval: Double.infinity) request.httpMethod = "" let task = URLSession.shared.dataTask(with: request) { data, response, error in guard let data = data else { print(String(describing: error)) return } print(String(data: data, encoding: .utf8)!) } task.resume()
// Example Dart Component var request = http.Request('', Uri.parse('https://perenual.com/api/hardiness-map?species_id=[ID]&key=[YOUR-API-KEY]')); http.StreamedResponse response = await request.send(); if (response.statusCode == 200) { print(await response.stream.bytesToString()); } else { print(response.reasonPhrase); }
// Example Kotlin Component val client = OkHttpClient() val request = Request.Builder() .url("https://perenual.com/api/hardiness-map?species_id=[ID]&key=[YOUR-API-KEY]") .post(RequestBody.create(null, ByteArray(0))) .build() val response = client.newCall(request).execute()
HELP & SUPPORT
Join our community of coders, get help & ask questions in a collaborative environment!😄
If you got any questions or help, feel free to contact us or reach us on Discord. If you would like to get started, click down below to get access to our API! Happy coding!